Requirements & Introduction
First we are gonna see what we want to achieve using matlab/octave (we can use any of this 2 IDE , i recommend using octave because is open source), we have to make sure also that we're using a "for loops" statement for the variables.
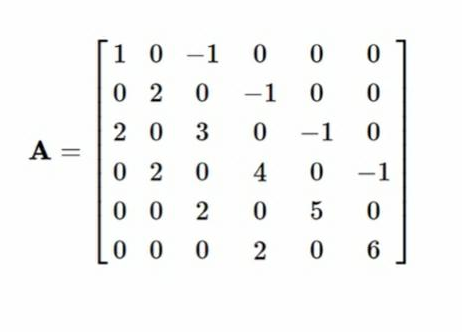
1° Step
Now that we know what is our goal, we are gonna start our code with naming our variables (we have to name 7 variables, 6 for our columns and rows, 1 for increment):
a=0;
b=0;
c=0;
d=0;
f=0;
g=0;
h=0;
2° Step
We add now the matriz full of zeros with this for function:
A = zeros(6,6);
Now we run our program, it should look like this (using the function "disp(A)"):
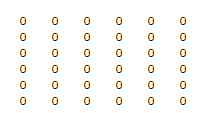
3° Step
We start coding our function for the diagonal numbers from 1 to 6:
for c = 1:6,
A(c,c) = ++h;
end
Now we run our program, it should look like this (using the function "disp(A)"):
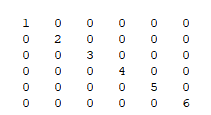
4° Step
Now we're gonna make the diagonal of 2's, now we know from the picture that the diagonal starts from the 3rd row and 1st column, from there it will automatically make 2's diagonally:
for d=1:4,
a=a+1;
b=b+1;
A(a,b)=2;
end
As you can see we use variables a & b and in our A(a,b) we already know it's (rows, columns), now we have to initialize a in 2, so now it can begin from 3rd row, we leave b as 0, so that way it starts from the 2nd row
a = 2;
b = 0;
It should look like this when we run it:
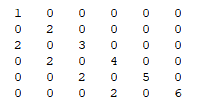
5° Step
5° and final step! We only need the diagonal of -1's, we use the same concept as step #5,
for e=1:4,
g=g+1;
f=f+1;
A(g,f)=-1;
end
As you can see we use variables g & f and in our A(g,f) we already know it's (rows, columns), now we have to initialize f in 2, so now it can begin from 3rd column, we leave g as 0, so that way it starts from the 1st row
g = 0;
f = 2;
disp(A)
Now our project should look like this: (don't forget to use "disp(A)")
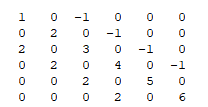
Full Code
I'll leave right here the code from my github, so you can take a good look at it!
Github Code